OVERVIEW
Problem: You have a Windows laptop at work, but want to develop Ansible playbooks using VS Code. Oh hey, VS Code has a cool Ansible plugin, so you install it and… it doesn’t work. You can’t take advantage of code-checking with ansible-lint, and you’re constantly shown errors like this:
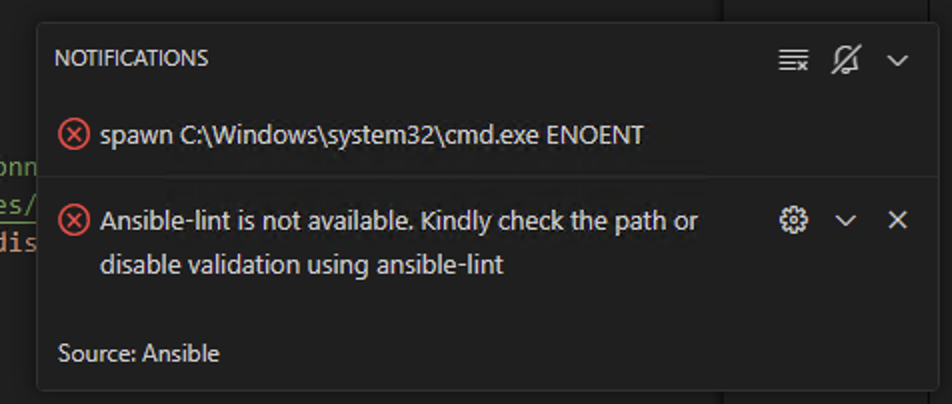
Well it turns out, the plugin relies on an Ansible executable to function, which is not available on Windows. Here on Red Hat’s blog, they break it down in simple terms: “For Windows users, the extension works with WSL2 and not on native Windows.”
So you have two options: Connect VS Code to a remote server or container running Linux, or connect it to a Linux OS locally via WSL2. This post is about the latter. Note that here we will use ‘WSL’ and ‘WSL2’ interchangeably.
If you don’t already know, WSL2 is the second iteration of Windows Subsystem for Linux (WSL), which essentially lets you run a bash terminal in a distro of your choice, in your Windows computer, without needing to spin up a dedicated virtual machine (VM). At least, not explicitly.
While you don’t need to open Hyper-V and configure a VM, that’s essentially what happens under the hood. From your perspective, you just launch the WSL application and boom, you’re in a bash shell. Behind the scenes, there is a really lightweight VM running the Linux kernel, but you don’t see it. You just get that sweet, sweet shell, in which you can use Linux tools, install Linux packages, and use all of the commands you’re used to. You can even work with files on your Windows computer, from that Linux shell.
Neat.
Out of the box, WSL offers several distros to choose from – the default is Ubuntu. But I work in a Red Hat shop, and while I am a Debian fan-boy, I’d like to use Red Hat 9 (RHEL9) in WSL, if for no other reason than to figure out how to do it (and be a Really Cool Guy TM in the process).
So I wrote this blog to document how I got it working. Mostly for future me, when he’s setting it up on another machine and forgot the details because it’s been longer than a week and the knowledge has long since vanished. Undoubtedly replaced in finite cranial storage by the ticket number of a cafe lunch order in 2009, or some other critically-irreplaceable life detail.
It’s pretty easy, although not without its gotchas. I’ll do my best to walk through the process, but keep in mind I am not an expert on WSL, this is just how I got it working initially for me.
NOTE: If your network security team does MITM / SSL inspection, you will have to get them to whitelist some URLs, or make some unsafe tweaks to /etc/pki/tls/openssl.cnf
that I won’t go into here.
The process of using a custom RHEL9 image in WSL boils down to 4 steps:
- Install WSL
- Import a custom RHEL9 image
- Configure the image
- Connect VS Code to WSL
We’re going to assume that #1 is already done. I also chose to make a directory specifically to hold my WSL images (which are basically just small .vhd files). For this example, I’m using C:\WSLImages
BUILD AND INSTALL THE IMAGE
So where do you get a RHEL9 image for WSL? You can build one in the customer console, give it an activation key and customize packages and repos, and then download it to your Windows machine. If you don’t have a paid Red Hat subscription, they do offer free developer accounts. You can use your developer account to build images and register your testing VMs, so they will be able to connect to Red Hat package repos.
I built a quick basic image, with no additional repos or packages, but with a preinstalled activation key. There are some packages you should include here, which I’ll install manually later on. What you end up with is a tar.gz file, that you don’t need to extract. The file will be named something like ‘composer-api-<GUID>.tar.gz.’
The following commands, run in Windows in an elevated command prompt or PowerShell, import the RHEL9 image and set it as the default distro. The default distro is the one that launches when WSL is executed.
#: wsl --import rhel9wsl C:\WSLimg\ C:\Users\coolguy\Downloads\composer-api-12345678-9a1b-2c3d-4e5f6g7h8i9j-disk.tar.gz
#: wsl --set-default rhel9wsl
Run ‘wsl’ or click on the icon to boot the image and you will get a root shell!
CONFIGURE THE IMAGE
By default, the WSL console has you automatically logged in as root – which is fine. This post on the Ubuntu forums does a good job of explaining why this is fine, namely:
- Your WSL instance runs with your Windows user’s permissions.
- Your Windows user has complete control over the Linux instance in WSL
So even running as root inside the WSL shell, and even though your C:\ drive is mounted to the Linux instance by default (at /mnt/c/), you won’t be able to modify anything on the Windows system that your Windows user couldn’t already do.
But even so, I prefer not to run as root if I don’t have to. So the first thing I do when I launch the WSL instance is change the root password and create a new user. I then set that user’s password and set it to log in by default (instead of root).
#: useradd <username>
#: usermod -aG wheel <username>
#: passwd <username>
To make your new user log in by default, add it to the [user]
section of /etc/wsl.conf
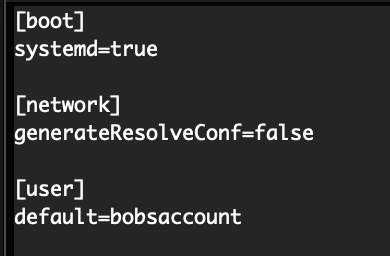
The generateResolvConf=false
bit prevents WSL from overriding your resolv.conf file at every startup. I set it this way so I could have it use my preferred DNS servers.
Reboot and reconnect. You should be logged in as the new user. Use ‘su’ to change back to root – there’s no sudo yet, so we have to install it later.
Now you’ll need to create your /etc/resolv.conf file.
My corporate network uses deep packet inspection, essentially a MITM to snoop SSL packets for nefarious contents. Which is fine, so long as the certificates it injects are valid and available. I made my best guess here, and downloaded the certificates from my browser’s store (search your settings for ‘certificates’). We use our own PKI, so what I got were two certificate files – one for the root CA and one for the issuing CA.
Don’t worry about what that means if it’s new vocabulary to you. Just get the certs and import them to the WSL distro like this:
#: cp /mnt/c/temp/my-rootca.cer /etc/pki/ca-trust/source/anchors/my-rootca.cer
#: cp /mnt/c/temp/my-issuingca.cer /etc/pki/ca-trust/source/anchors/my-issuingca.cer
#: update-ca-trust extract
Now you should be able to run subscription-manager register
without any SSL errors.
Now run dnf update
and then install these packages:
git
python3-pip
ansible-core
sudo
vim
wget
tmux
man
man-pages
Now reboot and re-lauch the WSL distro. You should be again automatically logged in to the user you created earlier. Only now you can sudo and all that cool stuff.
FINISHING TOUCHES
First things first. Create the various folders where you will clone your various Git repos:
$: mkdir /home/<user>/code/ORG1
$: mkdir /home/<user>/code/ORG2
Now you can install the Ansible linter with pip:
$: python3 -m pip install ansible-lint
Set up your git vars:
$: git config --global user.name "Ronald S."
$: git config --global user.email just_spam_me@hott-mails.net
I like to update my /etc/vimrc
as follows:
set number
set visualbell
set t_vb=
:color slate
set tabstop=4
set softtabstop=4
set shiftwidth=4
set expandtab
set autoindent
filetype plugin indent on
Also add this to your /etc/inputrc
and mute the CMD window in your Windows settings to silence all of the beeping.
set bell-style none
This is necessary for VS Code and the Ansible plugin. Create /etc/default/locale
and paste in this value (if you’re in the US, otherwise use your locale):
LANG=en_US.UTF-8
You’ll also want to add this to your ~/.bashrc
for the same reason.
export LC_ALL="C.UTF-8"
Don’t close bashrc yet. Add this for nice colors:
# Colors oooooh
PS1='\[\033[1;32m\]\u\[\033[1;37m\]@\[\033[1;36m\]\h\[\033[00m\]:\[\033[1;35m\]\w\[\033[00m\] \$ '
Now go ahead and save/close the ~/.bashrc file.
Load the new bash profile with source ~/.bashrc
and make sure everything works. The shell should look like this:
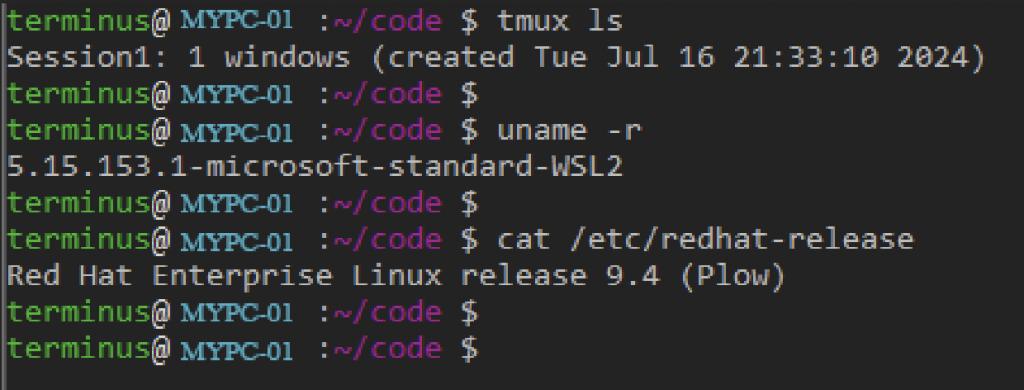
GO PLAY
Now you have a fully-functioning and registered Red Hat 9 image running in your Windows computer via WSL. Go play with it! Clone your git repos and connect VS Code to the Red Hat instance via the WSL plugin. WSL also integrates with Docker Desktop for Windows, so you can create and manage Docker containers from the Red Hat 9 shell. And you can run Linux GUI apps, and pin them to your Windows Start menu (like Wine, but in reverse?).
WSL has come a long way since it was first introduced some years ago. It’s no longer a nifty gimmick with niche use cases, it’s an essential part of your development environment if your workplace requires you to run a Windows workstation.
I hope you found this post at least slightly informative.